GitHub gives you a web-based hosting service for version control. It plays an essential role in software and web development. Nowadays, GitHub has more than 28 million users from all around the world. GitHub can be the best option for you if you want to allow users to log in with their social account besides the other social media OAuth Login (Facebook OAuth API, Google OAuth API, Twitter OAuth API, etc.). GitHub OAuth Login is a fast and powerful way to integrate the user login system in the web application.
The GitHub authentication API gives access to the users to sign in to the website using their GitHub account without registration on your website. Also, Log in with the GitHub account can be easily implemented using GitHub OAuth API. In this tutorial, we will explain to you how to integrate user login and registration system with GitHub by using PHP and store the user’s profile information in the MySQL database.
Before proceeding, let’s take a look at the file structure to implement GitHub Login with PHP.
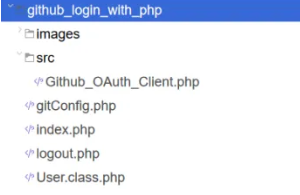
Create a GitHub OAuth Application
You need to create and register the OAuth App to access GitHub API. Also, you need to specify the Client ID & Client Secret at the time of the GitHub API call. Follow the steps given below to create and configure a GitHub OAuth application.
- Log in to your GitHub account. In the upper corner on the right side, click your profile image and then click Settings.

- Now, in the settings page, click Developer settings on the left sidebar.
- In the left sidebar, click OAuth Apps. To create a new OAuth app, you have to click the Register a new application.

- Enter all the necessary information (Application name, URL Homepage, Application description, and Authorization callback URL) to register your new OAuth application. Click the Register application when you’re done.
the Register application - Generate application of the Client ID and Client Secret. Copy the GitHub OAuth information (Client ID and Client Secret)to use it later the script to access GitHub API.
Create Database Table
If you want to store the user’s profile information from the GitHub account, you have to create a table in the database. The following SQL creates a user table with some primary fields in the MySQL database.
CREATE TABLE `users` ( `id` int(11) NOT NULL AUTO_INCREMENT, `oauth_provider` enum('','github','facebook','google','twitter') COLLATE utf8_unicode_ci NOT NULL, `oauth_uid` varchar(100) COLLATE utf8_unicode_ci NOT NULL, `name` varchar(50) COLLATE utf8_unicode_ci NOT NULL, `username` varchar(50) COLLATE utf8_unicode_ci NOT NULL, `email` varchar(100) COLLATE utf8_unicode_ci NOT NULL, `location` varchar(100) COLLATE utf8_unicode_ci DEFAULT NULL, `picture` varchar(255) COLLATE utf8_unicode_ci NOT NULL, `link` varchar(255) COLLATE utf8_unicode_ci NOT NULL, `created` datetime NOT NULL, `modified` datetime NOT NULL, PRIMARY KEY (`id`) ) ENGINE=InnoDB DEFAULT CHARSET=utf8 COLLATE=utf8_unicode_ci;
Github OAuth Client for PHP (Github_OAuth_Client.php)
The Github_OAuth_Client is a PHP library. It supports OAuth for GitHub REST API.
- getAuthorizeURL() – Generate URL to authorize with the Github account.
- get access token() – Exchange OAuth code and gain access token from Github OAuth API.
- API request() – Make an API call and retrieve the access token or user’s account data using from Github OAuth API.
<?php /* * Class Github_OAuth_Client * * Author: CodexWorld * Author URL: https://www.codexworld.com * Author Email: [email protected] * * The first PHP Library to support OAuth for GitHub REST API. */ class Github_OAuth_Client{ public $authorizeURL = "https://github.com/login/oauth/authorize"; public $tokenURL = "https://github.com/login/oauth/access_token"; public $apiURLBase = "https://api.github.com/"; public $clientID; public $clientSecret; public $redirectUri; /** * Construct object */ public function __construct(array $config = []){ $this->clientID = isset($config['client_id']) ? $config['client_id'] : ''; if(!$this->clientID){ die('Required "client_id" key not supplied in config'); } $this->clientSecret = isset($config['client_secret']) ? $config['client_secret'] : ''; if(!$this->clientSecret){ die('Required "client_secret" key not supplied in config'); } $this->redirectUri = isset($config['redirect_uri']) ? $config['redirect_uri'] : ''; } /** * Get the authorize URL * * @returns a string */ public function getAuthorizeURL($state){ return $this->authorizeURL . '?' . http_build_query([ 'client_id' => $this->clientID, 'redirect_uri' => $this->redirectUri, 'state' => $state, 'scope' => 'user:email' ]); } /** * Exchange token and code for an access token */ public function getAccessToken($state, $oauth_code){ $token = self::apiRequest($this->tokenURL . '?' . http_build_query([ 'client_id' => $this->clientID, 'client_secret' => $this->clientSecret, 'state' => $state, 'code' => $oauth_code ])); return $token->access_token; } /** * Make an API request * * @return API results */ public function apiRequest($access_token_url){ $apiURL = filter_var($access_token_url, FILTER_VALIDATE_URL)?$access_token_url:$this->apiURLBase.'user?access_token='.$access_token_url; $context = stream_context_create([ 'http' => [ 'user_agent' => 'CodexWorld GitHub OAuth Login', 'header' => 'Accept: application/json' ] ]); $response = @file_get_contents($apiURL, false, $context); return $response ? json_decode($response) : $response; } }
User Class (User.class.php)
The User class handles the database related operations (fetch, update, and insert). Make sure the database host ($dbHost), username ($dbUsername), password ($dbPassword), and name ($dbName) as per your MySQL database credentials.
- __construct() – Connect to the database.
- check user() – Check whether the user data already exists in the database. Update if user data exists, otherwise insert the user’s profile data in the user’s table using PHP and MySQL.
<?php /* * Class User * * Author: CodexWorld * Author URL: https://www.codexworld.com * Author Email: [email protected] * * Handles database related works */ class User { private $dbHost = "localhost"; private $dbUsername = "root"; private $dbPassword = "root"; private $dbName = "codexworld"; private $userTbl = 'users'; function __construct(){ if(!isset($this->db)){ // Connect to the database $conn = new mysqli($this->dbHost, $this->dbUsername, $this->dbPassword, $this->dbName); if($conn->connect_error){ die("Failed to connect with MySQL: " . $conn->connect_error); }else{ $this->db = $conn; } } } function checkUser($userData = array()){ if(!empty($userData)){ // Check whether user data already exists in database $prevQuery = "SELECT * FROM ".$this->userTbl." WHERE oauth_provider = '".$userData['oauth_provider']."' AND oauth_uid = '".$userData['oauth_uid']."'"; $prevResult = $this->db->query($prevQuery); if($prevResult->num_rows > 0){ // Update user data if already exists $query = "UPDATE ".$this->userTbl." SET name = '".$userData['name']."', username = '".$userData['username']."', email = '".$userData['email']."', location = '".$userData['location']."', picture = '".$userData['picture']."', link = '".$userData['link']."', modified = NOW() WHERE oauth_provider = '".$userData['oauth_provider']."' AND oauth_uid = '".$userData['oauth_uid']."'"; $update = $this->db->query($query); }else{ // Insert user data $query = "INSERT INTO ".$this->userTbl." SET oauth_provider = '".$userData['oauth_provider']."', oauth_uid = '".$userData['oauth_uid']."', name = '".$userData['name']."', username = '".$userData['username']."', email = '".$userData['email']."', location = '".$userData['location']."', picture = '".$userData['picture']."', link = '".$userData['link']."', created = NOW(), modified = NOW()"; $insert = $this->db->query($query); } // Get the user data from the database $result = $this->db->query($prevQuery); $userData = $result->fetch_assoc(); } // Return the user data return $userData; } }
Github OAuth API Configuration (gitConfig.php)
In the gitConfig.php file, initialize the Github OAuth PHP Client library to connect with Github API and working with SDK. Specify the client ID ($clientID) and client secret ($clientSecret) as per your GitHub OAuth application. Besides, specify the redirect URL ($redirectURL) as per your script location.
<?php // Start session if(!session_id()){ session_start(); } // Include Github client library require_once 'src/Github_OAuth_Client.php'; /* * Configuration and setup GitHub API */ $clientID = 'Your_App_Client_ID'; $clientSecret = 'Your_App_Client_Secret'; $redirectURL = 'https://www.codexworld.com/github-login/'; $gitClient = new Github_OAuth_Client(array( 'client_id' => $clientID, 'client_secret' => $clientSecret, 'redirect_uri' => $redirectURL, )); // Try to get the access token if(isset($_SESSION['access_token'])){ $accessToken = $_SESSION['access_token']; }
Login & Fetch GitHub Profile Data (index.php)
If the user is already logged in with a GitHub account, the profile information (account ID, name, email, location, username, profile picture, and profile link) will be displayed. Otherwise, the GitHub login button will appear.
- If the access token already exists in the SESSION, the following things will happen:
- The user profile data is secured from Github using the API request() function of Github_OAuth_Client class.
- Pass profile data in the checkUser() function to insert or update user data in the database.
- Store the user account data in the SESSION.
- Show Github profile data to the user.
- If the code parameter is present in the query string of the URL, the following occurs:
- Verify the state whether it matches with the stored state.
- Now, get an access token using get access token() function of Github_OAuth_Client class.
- Store access token in the SESSION.
- Or else:
- Generate an unguessable random string and store it in the SESSION.
- Again, get the authorize URL using the getAuthorizeURL() function of Github_OAuth_Client class.
- Render a Github login button on the web page.
<?php // Include GitHub API config file require_once 'gitConfig.php'; // Include and initialize user class require_once 'User.class.php'; $user = new User(); if(isset($accessToken)){ // Get the user profile info from Github $gitUser = $gitClient->apiRequest($accessToken); if(!empty($gitUser)){ // User profile data $gitUserData = array(); $gitUserData['oauth_provider'] = 'github'; $gitUserData['oauth_uid'] = !empty($gitUser->id)?$gitUser->id:''; $gitUserData['name'] = !empty($gitUser->name)?$gitUser->name:''; $gitUserData['username'] = !empty($gitUser->login)?$gitUser->login:''; $gitUserData['email'] = !empty($gitUser->email)?$gitUser->email:''; $gitUserData['location'] = !empty($gitUser->location)?$gitUser->location:''; $gitUserData['picture'] = !empty($gitUser->avatar_url)?$gitUser->avatar_url:''; $gitUserData['link'] = !empty($gitUser->html_url)?$gitUser->html_url:''; // Insert or update user data to the database $userData = $user->checkUser($gitUserData); // Put user data into the session $_SESSION['userData'] = $userData; // Render Github profile data $output = '<h2>Github Profile Details</h2>'; $output .= '<img src="'.$userData['picture'].'" />'; $output .= '<p>ID: '.$userData['oauth_uid'].'</p>'; $output .= '<p>Name: '.$userData['name'].'</p>'; $output .= '<p>Login Username: '.$userData['username'].'</p>'; $output .= '<p>Email: '.$userData['email'].'</p>'; $output .= '<p>Location: '.$userData['location'].'</p>'; $output .= '<p>Profile Link : <a href="'.$userData['link'].'" target="_blank">Click to visit GitHub page</a></p>'; $output .= '<p>Logout from <a href="logout.php">GitHub</a></p>'; }else{ $output = '<h3 style="color:red">Some problem occurred, please try again.</h3>'; } }elseif(isset($_GET['code'])){ // Verify the state matches the stored state if(!$_GET['state'] || $_SESSION['state'] != $_GET['state']) { header("Location: ".$_SERVER['PHP_SELF']); } // Exchange the auth code for a token $accessToken = $gitClient->getAccessToken($_GET['state'], $_GET['code']); $_SESSION['access_token'] = $accessToken; header('Location: ./'); }else{ // Generate a random hash and store in the session for security $_SESSION['state'] = hash('sha256', microtime(TRUE) . rand() . $_SERVER['REMOTE_ADDR']); // Remove access token from the session unset($_SESSION['access_token']); // Get the URL to authorize $loginURL = $gitClient->getAuthorizeURL($_SESSION['state']); // Render Github login button $output = '<a href="'.htmlspecialchars($loginURL).'"><img src="images/github-login.png"></a>'; } ?> <!DOCTYPE html> <html lang="en-US"> <head> <title>Login with GitHub using PHP by CodexWorld</title> <meta charset="utf-8"> </head> <body> <div class="container"> <!-- Display login button / GitHub profile information --> <div class="wrapper"><?php echo $output; ?></div> </div> </body> </html>
Logout (logout.php)
The logout.php file logs the user out from their Github account.
- Unset all the SESSION variables (access_token, state, and userData) in PHP.
- Redirect the user to the homepage.
<?php // Start session if(!session_id()){ session_start(); } // Remove access token and state from session unset($_SESSION['access_token']); unset($_SESSION['state']); // Remove user data from session unset($_SESSION['userData']); // Redirect to the homepage header("Location:index.php"); ?>
Conclusion
Our GitHub OAuth PHP library helps integrate login with Github in PHP. Firstly, you can quickly implement the Github OAuth login system in a web application. Secondly, the example code functionality can be extended as per the requirement of your application. Besides, we also recommend adding other social accounts to the PHP login system to provide you with a user-friendly way so that the user can log in to your website.